Because that Matlab is such a powerful interactive and programming tool, it's easy to add functionality when you think of a new need. For example, yesterday I ran for 36 minutes, divided into a 10 min easy warm-up, and 26 mins at pace. The Nike+ website gives me:
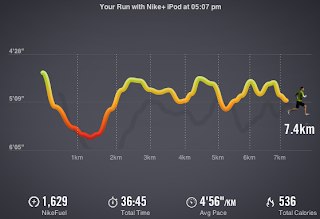
which doesn't quite reflect the nature of my workout. Instead, what I want is the following:
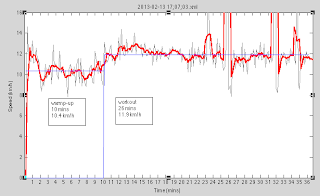
This graph makes a lot more sense. You can see I have marked the average speed for both the first 10 mins (10.4 km/h) and the subsequent section (11.9 km/h) separately. I have overlaid the plot (raw and smooth) with the averages for the two sections as horizontal lines in blue.
To plot the recorded data, I simply call the nike function from last time:
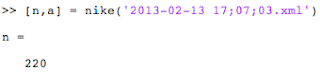
Note, I've modified the call (and code) a bit to return both the number of data points collected and the array itself. (This is because I'm going to massage the data a bit.) At this point, I notice that there are a few blips in the data. There are two recorded data points that say I'm running at 60 and 80 km/h, respectively - which of course, is absurd. These data points will affect the average speed recorded, so I'll want to remove them by running another function that I wrote for the purpose, called rmoutliers:
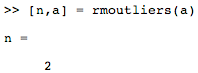
It returns n, the number of outliers detected, and the modified array (a). Here, n=2 and all is well. How did I define what constitutes an outlier? Well, I crudely decided that 3 standard deviations away from the mean would count. Here is the code:
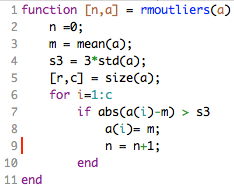
Notice I simply decided to replace those outlier points with the mean. (I could do something different and a bit more realistic, like use a moving average, of course.)
Finally, I want to overlay my average speed for the first 10 minutes and the rest of the run. We can add another function plotavg as follows:
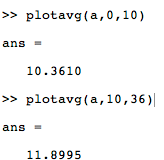
I wrote this to say plot the average for mins 0 through 10. And then the average for mins 10 through 36. And in both cases, tell me what the average was for the defined segment. The code is very simple:
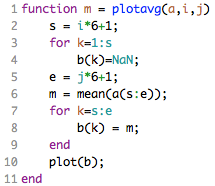
The end result is:
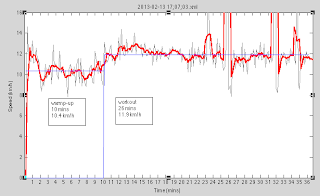
This functionality is simply not possible using a program with a simple, fixed menu of options because there will always be more things you'd like the program to have that you didn't think of initially. And one might be reluctant to dig into code and recompile an updated application just for one more function. But because Matlab is so programmable, these tasks are easy to do even on one-time basis.